Google Apps Script:
The Fundamentals
A primer of things remarkably difficult to find in the official documentation!
Javascript plus Google Services
Google Apps Scripts are written in standard JavaScript (executed using the V8 runtime), supplemented with “Apps Script services” that automate interaction with Google Apps (Sheets, Forms, Classroom, etc). These exist as global objects with associated methods. Most are built-in by default, but some “advanced services” need to be added in using the script editor.
A full reference for all of these is provided, but this isn’t always entirely clear to the ethusiastic ametuer. This site aims to fill in the gaps, in particular by offering commonly needed code snippets.
The script editor
Apps Script has a built-in editor which you can access from within apps by clicking Extensions > Apps Script or directly at script.google.com.
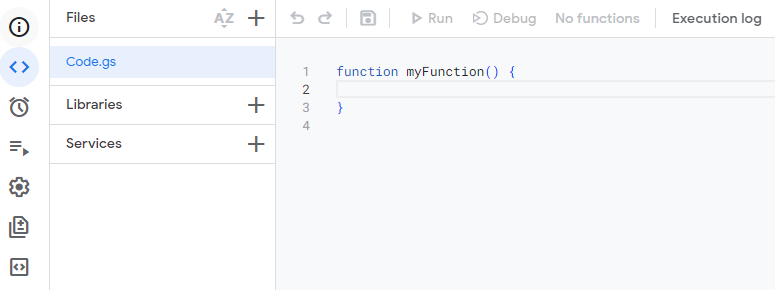
You might already have a favourite code editor, but you won’t need it for writing Apps Script code. Uploading externally-edited code isn’t straightforward and, more importantly, the in-built editor autocompletes all of the Apps Script service objects and methods. It is also where you can add any advanced services you need and it lists all of the related files for your Apps Script project.
If you want to customise the editor, try the Black Apps Script Chrome add-on.
The editor can only open one script file at a time, but you can open multiple editor windows in your browser at the same time.
Container-bound (as opposed to stand-alone) scripts
If you open the editor from within an app (Extensions menu > Apps Script), the script you write will be “container-bound” to the open file. This means the script doesn’t appear as a separate file in Google Drive (although it can be accessed via script.google.com); it is part of the Sheets, Docs, Slides, Forms file (the “container”) and it can’t be detached.
The notable difference compared to standalone Apps Script projects is that container-bound projects are able to add custom menus (to launch your functions) and show dialogs and sidebars (for interacting with the user). They can also reference their parent file without needing to use the file ID. Most, if not all, of your scripts are going to be container-bound.
If you want to copy a container-bound script, you must copy and paste the text to another editor window.
Script files (.gs)
Your script is saved in the editor as “.gs” files, visible in the Files column on the left hand side of the editor window.
It is good practice to divide long code into separate files for different aspects of your Apps Scripts “project”.
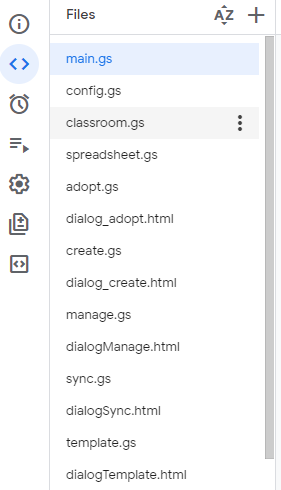
(Dialogs are built from small HTML files. These will also appear in the project’s file list.)
Placing the code into separate files just makes it easier to read and maintain; when you run the script everything is “parsed” as if it was all in a single file.
Running your script
You can execute your script in different ways, including:
- Automatically via a “project trigger”, e.g. the container file being opened, a cell being edited, a form being submitted.
- By clicking an option in a custom menu that your script creates.
- By clicking “Run” in the script editor.
- By creating a custom function that works in Sheets when you type “=yourFunctionName”, just like built-in functions.
Server-side execution
When your script runs, it runs on Google’s servers, not your computer. Google places limits on this. In particular, no script can take longer than 6 minutes to complete.
Multiple instances
One of the most crucial things to understand about Google Apps Script is that every time any aspect of your script is called to run, the entire script is executed completely afresh. There is no “memory”. Anything that happened in a previous run is forgotten unless the outcome is saved somewhere (e.g. a cell on the container Sheet).
Parsing
Parsing is the process of turning your human-readable code into a format that the “runtime environment” can actually run.
The thing to understand here is that Apps Script files are just like any other JavaScript files. In particular, your code doesn’t have to exist only inside functions. You might conclude otherwise given that when you open a new project the editor presents you with a new myFunction()
to fill in and all of Google’s example code snippets only exist within functions.
Any variables you place outside of a function have global scope and can be accessed by all functions. Remember, though, that every execution of the script is a new execution and so the values of these “globals” will have their values reset when, for instance, the user restarts the script by selecting from the custom menu.
delete
methods
In the services reference, some objects have delete
methods. The reference is written for API developers rather than Apps Script coders, however. This is important to know, because delete
is a reserved word in JavaScript and so in Apps Script all delete
methods are renamed to remove
!
Requests and responses
Most service methods take arguments referred to as the “request body” and they return a “response”. The reference for the method indicates what form each of these take. For example:
Useful resources
A primer of things remarkably difficult to find in the official documentation!